In order to install realm, requires as follows, in build.gradle (:app)
apply plugin: 'realm-android'
in build.gradle (:Project level)
buildscript {
ext.realm_version = '10.15.1'
repositories {
google()
maven { url "https://github.com/realm/realm-java" } // Realm repository
}
dependencies {
classpath 'com.android.tools.build:gradle:8.3.1'
classpath "io.realm:realm-gradle-plugin:$realm_version"
classpath "io.realm:realm-gradle-plugin:$realm_version-transformer-api"
classpath "io.realm:realm-gradle-plugin:10.15.1" // Use the latest version
}
}
What Each Line Does:
- Android Gradle Plugin (
com.android.tools.build:gradle
): Required for building Android projects.- Realm Gradle Plugin (
io.realm:realm-gradle-plugin
): Integrates Realm into the build process, enabling schema management and other Realm features.- Transformer API (
io.realm:realm-gradle-plugin:$realm_version-transformer-api
):
- Adds support for advanced features like Realm class transformations (only required in certain cases).
- Explicit Version (
io.realm:realm-gradle-plugin:10.15.1
):
- Ensures the latest version is used directly (useful for debugging or ensuring compatibility).
How it Works Together
Project-Level Setup:
- The
build.gradle (:Project level)
file configures the build environment with the necessary dependencies and repositories to use Realm. - It ensures the Realm Gradle plugin is available during the build process.
- The
Module-Level Plugin:
- The
build.gradle (:app)
file applies therealm-android
plugin to enable Realm features for your app.
- The
Compilation and Integration:
- During the build, the Realm Gradle plugin processes your model classes and generates the necessary schema code for the Realm database.
- The plugin also ensures that Realm’s libraries and dependencies are included in your APK.
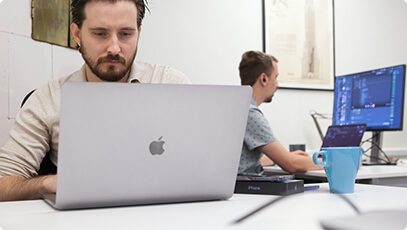
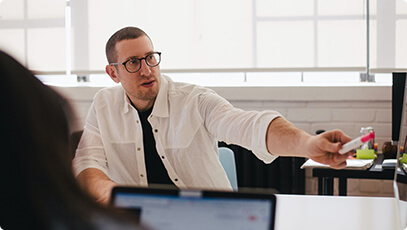
Simplified Version
Here’s a streamlined version of your configuration:
Project-Level build.gradle
buildscript {
ext.realm_version = '10.15.1'
repositories {
google()
maven { url "https://github.com/realm/realm-java" }
}
dependencies {
classpath 'com.android.tools.build:gradle:8.3.1'
classpath "io.realm:realm-gradle-plugin:$realm_version"
}
}
App-Level build.gradle
apply plugin: 'realm-android'
This configuration should be sufficient for most cases unless advanced features are needed.